Functional Interfaces in Java
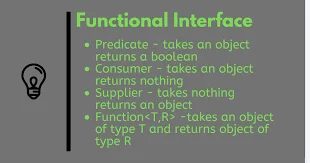
Introduction to Functional Interfaces
Functional interfaces, a core concept in modern Java development, are interfaces that contain exactly one abstract method, known as the functional method. This characteristic distinguishes them from other interfaces, which can have multiple abstract methods. The single abstract method in a functional interface represents the primary purpose or action that the interface is designed to perform.
The introduction of functional interfaces was a significant enhancement in Java 8, aligning Java with other functional programming languages. Their main role is to facilitate the use of lambda expressions and method references, enabling more concise and readable code. The functional method in these interfaces serves as a target for lambda expressions, promoting a more functional approach to coding in Java.
Despite having only one abstract method, functional interfaces can still include default and static methods. These methods provide implementations that do not interfere with the functional method’s singularity, thus preserving the interface’s functional nature. The inclusion of default and static methods offers flexibility and additional functionality without compromising the simplicity and clarity of the interface’s primary purpose.
Examples of functional interfaces in the Java standard library include java.util.function.Predicate
, java.util.function.Function
, and java.util.function.Consumer
. These interfaces are widely used in stream operations and other functional programming constructs within the Java ecosystem. By adhering to the principle of having one abstract method, they allow developers to leverage functional programming paradigms effectively.
Understanding functional interfaces is crucial for grasping the full potential of Java’s functional programming capabilities. As we delve deeper into their significance and usage, it becomes evident how they streamline code, enhance readability, and facilitate the implementation of complex logic in a more manageable and intuitive manner.
Examples of Built-in Functional Interfaces
Java provides several built-in functional interfaces that are essential for functional programming. Two of the most frequently used examples are Runnable
and Comparator
. These interfaces facilitate the implementation of lambda expressions and method references, thereby enhancing code readability and efficiency.
The Runnable
interface is perhaps the most straightforward functional interface in Java. It contains a single abstract method, run()
, which does not accept any parameters and does not return a value. This makes it ideal for defining tasks that can be executed by a thread. For example:
Runnable task = () -> System.out.println("Task is running");Thread thread = new Thread(task);thread.start();
In the code above, a lambda expression is used to implement the run()
method of the Runnable
interface. This task is then executed by a new thread, showcasing the simplicity and effectiveness of using functional interfaces.
The Comparator
interface is another widely used functional interface, primarily for comparing objects. It contains the abstract method compare(T o1, T o2)
, which takes two objects of the same type and returns an integer. This method allows for custom sorting logic. For instance:
Comparator stringComparator = (s1, s2) -> s1.compareTo(s2);List<String> names = Arrays.asList("John", "Jane", "Adam", "Eve");Collections.sort(names, stringComparator);
In this example, a lambda expression is used to implement the compare()
method. The Comparator
then sorts a list of names in natural order. This demonstrates how functional interfaces like Comparator
can simplify and streamline operations that involve custom comparison logic.
Understanding and utilizing built-in functional interfaces such as Runnable
and Comparator
can significantly enhance the expressiveness and efficiency of Java code. By leveraging these interfaces, developers can write more concise and maintainable programs.
Introduction of Default Methods in Java 8
With the release of Java 8, the concept of default methods was introduced, marking a significant evolution in the language. Default methods, also known as defender methods or virtual extension methods, allow developers to add new methods to interfaces without breaking the existing implementation of classes that already implement these interfaces. This capability provides enhanced flexibility in interface design and evolution.
Default methods are defined using the default
keyword within an interface. They can have a body just like regular class methods, enabling them to provide a default implementation. This feature is particularly useful when evolving interfaces, as it allows new methods to be introduced without requiring all implementing classes to provide an implementation. Here is an example of how a default method can be added to an interface:
public interface MyInterface {
void abstractMethod();
default void defaultMethod() {
System.out.println("This is a default method.");
}
}
While interfaces can include multiple default methods, it is crucial to note that a functional interface is still restricted to having just one abstract method. This single abstract method criterion is what defines a functional interface, ensuring it can be utilized in lambda expressions and method references. The addition of default methods does not alter this fundamental characteristic of functional interfaces.
In summary, default methods introduced in Java 8 provide a means to enhance interfaces with new functionalities while maintaining backward compatibility. This feature allows developers to evolve their codebase more smoothly. However, it is essential to remember that despite the addition of multiple default methods, a functional interface must adhere to having only one abstract method.
Defining Default Methods in Functional Interfaces
Default methods in functional interfaces allow developers to add new functionality to interfaces without breaking existing implementations. This feature, introduced in Java 8, ensures backward compatibility while enabling the evolution of interfaces. A default method is defined using the default
keyword followed by the method body, which provides a concrete implementation. Here is a basic syntax example of a default method in a functional interface:
public interface MyFunctionalInterface {void abstractMethod();default void defaultMethod() {System.out.println("This is a default method.");}}
In this example, MyFunctionalInterface
contains an abstract method named abstractMethod
, adhering to the single abstract method requirement. The defaultMethod
provides additional functionality without the need for implementation in the classes that use this interface. These default methods can be particularly useful when extending an interface to add new methods without forcing all implementing classes to update.
A practical use case for default methods can be seen in the evolution of large-scale APIs. Consider the List
interface in the Java Collections Framework. Prior to Java 8, any new method added to this interface would require all implementing classes to provide an implementation. With default methods, new functionalities such as sort
could be added without breaking the existing codebase:
public interface List extends Collection {void add(E e);E get(int index);default void sort(Comparator c) {Collections.sort(this, c);}}
The sort
method in the List
interface is a default method that leverages the Collections.sort
implementation. By utilizing default methods, developers can ensure that interfaces remain extensible and maintainable. They provide a powerful mechanism for enhancing the capabilities of functional interfaces while preserving their core simplicity.
The @FunctionalInterface Annotation
The @FunctionalInterface
annotation, introduced in Java 8, serves a crucial role in defining and enforcing the concept of functional interfaces. A functional interface is an interface that contains only one abstract method, making it suitable for use with lambda expressions and method references. By annotating an interface with @FunctionalInterface
, developers can explicitly declare their intent that the interface is meant to be a functional interface.
Using the @FunctionalInterface
annotation offers several benefits. Firstly, it provides documentation and clarity, making the code more readable and understandable. When another developer or a future version of yourself revisits the code, the annotation immediately signals that the interface is intended to be functional, with only one abstract method.
Secondly, the @FunctionalInterface
annotation enforces a single abstract method rule at compile time. If an interface marked with this annotation contains more than one abstract method, the compiler will generate an error, thereby preventing accidental addition of extra abstract methods. This ensures that the interface remains compatible with lambda expressions and method references, maintaining its functional nature.
To use the @FunctionalInterface
annotation, one simply needs to place it above the interface declaration. For example:
@FunctionalInterfacepublic interface MyFunctionalInterface {void execute();}
In this example, MyFunctionalInterface
is marked as a functional interface with a single abstract method execute()
. If an attempt is made to add another abstract method, the compiler will flag it as an error, preserving the functional interface integrity.
In conclusion, the @FunctionalInterface
annotation is a powerful tool in Java that not only documents the intent of an interface but also enforces the rules of functional interfaces. By leveraging this annotation, developers can write more robust, clear, and functional code, ensuring compatibility with lambda expressions and method references.
Lambda Expressions and Functional Interfaces
In Java, the concept of functional interfaces is tightly interwoven with lambda expressions. A functional interface is an interface with a single abstract method, and it can be implemented using a lambda expression. This combination brings a new level of expressiveness and conciseness to Java code, simplifying development and enhancing readability.
Lambda expressions provide a clear and concise way to represent the implementation of a functional interface. By using lambda expressions, developers can eliminate much of the boilerplate code that traditionally accompanies anonymous inner classes. This not only streamlines the code but also makes it more readable and maintainable.
Consider a scenario where you have a functional interface with a single method. Traditionally, implementing this interface would require creating an anonymous inner class. However, with lambda expressions, you can achieve the same implementation in a much more succinct manner. For instance, if you have a functional interface Calculator
with a method calculate(int a, int b)
, it can be implemented using a lambda expression like this:
Calculator addition = (a, b) -> a + b;
This single line of code replaces several lines of boilerplate that would have been necessary with an anonymous inner class. The lambda expression `(a, b) -> a + b;` succinctly defines the method’s implementation, making the code more intuitive and easier to follow.
The synergy between lambda expressions and functional interfaces also empowers developers to employ functional programming paradigms within Java. This shift allows for more declarative programming styles, where the focus is on the ‘what’ rather than the ‘how’. As a result, code becomes more expressive and less error-prone.
Overall, the integration of lambda expressions with functional interfaces in Java serves to simplify code, enhance readability, and promote a more functional approach to programming. This powerful combination is a significant advancement in the Java language, offering developers a more efficient and streamlined coding experience.
Common Pitfalls and Best Practices
When working with functional interfaces in Java, developers often encounter several common pitfalls that can lead to inefficiencies and errors. One frequent mistake is the improper use of lambda expressions. While lambda expressions provide a concise way to implement functional interfaces, they can become problematic if not used judiciously. For example, overly complex lambda expressions can reduce code readability and make debugging more challenging. To avoid this, keep lambda expressions simple and readable, adhering to the single-responsibility principle.
Another common mistake is neglecting the use of the @FunctionalInterface
annotation. This annotation is not mandatory, but it is highly recommended as it ensures that the interface meets the criteria of a functional interface. By explicitly declaring an interface as a functional interface, you make your intentions clear and enable the compiler to enforce the contract, thus preventing accidental addition of multiple abstract methods.
In addition, developers sometimes overlook the importance of type inference in lambda expressions. While type inference can simplify code, it can also lead to ambiguity and unexpected behavior if not handled correctly. Ensure that the types are explicitly stated when ambiguity might arise, especially in complex expressions or when dealing with overloaded methods.
On the side of best practices, it is crucial to follow the principle of immutability. Functional programming paradigms advocate for immutability to maintain consistency and predictability in code. When designing functional interfaces, ensure that the methods do not modify the state of the objects. Instead, return new instances with the updated state.
Furthermore, take advantage of the built-in functional interfaces provided by the Java standard library, such as Function
, Predicate
, and Consumer
. These interfaces cover a wide range of common scenarios and can save time and effort in defining custom ones. Utilizing these standard interfaces also makes your code more consistent and easier to understand for other developers.
Lastly, remember to document your functional interfaces thoroughly. Clear documentation helps other developers understand the purpose and usage of the interface, reducing the likelihood of misuse. By adhering to these best practices and avoiding common pitfalls, you can ensure that your functional interfaces remain clean, efficient, and aligned with Java’s functional programming paradigms.
Conclusion and Future Trends
In this comprehensive exploration of functional interfaces in Java, we have delved into their fundamental role within the language’s ecosystem. Functional interfaces, by serving as the cornerstone of Java’s approach to functional programming, enable developers to write more concise, readable, and maintainable code. By leveraging the power of lambda expressions and method references, programmers can simplify complex logic and enhance the flexibility of their applications.
Functional interfaces are pivotal in modern Java programming, underpinning many of the language’s advanced features such as the Stream API and the Optional class. Their integration allows for a more declarative style of programming, which is increasingly favored in contemporary software development due to its clarity and efficiency. The adoption of functional programming paradigms in Java not only modernizes the language but also aligns it with current industry trends and best practices.
Looking ahead, future advancements in Java are likely to further embrace and extend the principles of functional programming. Upcoming versions of the language may introduce new functional interfaces or enhancements to existing ones, providing developers with even more powerful tools to express complex operations succinctly. Additionally, there is potential for greater synergy between Java and other functional programming languages, leading to a more cohesive and versatile development environment.
As the landscape of software development continues to evolve, the importance of functional interfaces in Java cannot be overstated. They represent a critical bridge between object-oriented and functional programming paradigms, offering a pragmatic approach to leveraging the strengths of both. Developers who master functional interfaces will be well-equipped to tackle the challenges of modern software engineering, ensuring their code is both robust and adaptable to future innovations.